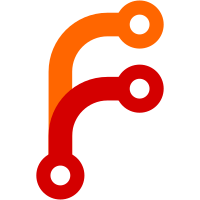
Notifications are not persistent, and once gone there is no way to accept the request. Clicking the notification in the notification history does nothing in plasma and gnome. There make them persistent and close them after the pairing timeout, after which they are no longer useful. BUG: https://phabricator.kde.org/T5002
93 lines
3.4 KiB
C++
93 lines
3.4 KiB
C++
/**
|
|
* Copyright 2014 Yuri Samoilenko <kinnalru@gmail.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License or (at your option) version 3 or any later version
|
|
* accepted by the membership of KDE e.V. (or its successor approved
|
|
* by the membership of KDE e.V.), which shall act as a proxy
|
|
* defined in Section 14 of version 3 of the license.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <QApplication>
|
|
#include <QNetworkAccessManager>
|
|
#include <QTimer>
|
|
|
|
#include <KDBusService>
|
|
#include <KNotification>
|
|
#include <KLocalizedString>
|
|
#include <KIO/AccessManager>
|
|
|
|
#include "core/daemon.h"
|
|
#include "core/device.h"
|
|
#include "core/backends/pairinghandler.h"
|
|
#include "kdeconnect-version.h"
|
|
|
|
class DesktopDaemon : public Daemon
|
|
{
|
|
Q_OBJECT
|
|
Q_CLASSINFO("D-Bus Interface", "org.kde.kdeconnect.daemon")
|
|
public:
|
|
DesktopDaemon(QObject* parent = Q_NULLPTR)
|
|
: Daemon(parent)
|
|
, m_nam(Q_NULLPTR)
|
|
{}
|
|
|
|
void askPairingConfirmation(PairingHandler* d) override
|
|
{
|
|
KNotification* notification = new KNotification(QStringLiteral("pairingRequest"),
|
|
KNotification::Persistent);
|
|
notification->setIconName(QStringLiteral("dialog-information"));
|
|
notification->setComponentName(QStringLiteral("kdeconnect"));
|
|
notification->setText(i18n("Pairing request from %1", getDevice(d->deviceLink()->deviceId())->name()));
|
|
notification->setActions(QStringList() << i18n("Accept") << i18n("Reject"));
|
|
connect(notification, &KNotification::ignored, d, &PairingHandler::rejectPairing);
|
|
connect(notification, &KNotification::action1Activated, d, &PairingHandler::acceptPairing);
|
|
connect(notification, &KNotification::action2Activated, d, &PairingHandler::rejectPairing);
|
|
QTimer::singleShot(d->pairingTimeoutMsec(), notification, &KNotification::close); // close after pairing timeout, assuming that the peer uses the same timeout value
|
|
notification->sendEvent();
|
|
}
|
|
|
|
void reportError(const QString & title, const QString & description) override
|
|
{
|
|
KNotification::event(KNotification::Error, title, description);
|
|
}
|
|
|
|
QNetworkAccessManager* networkAccessManager() override
|
|
{
|
|
if (!m_nam) {
|
|
m_nam = new KIO::AccessManager(this);
|
|
}
|
|
return m_nam;
|
|
}
|
|
|
|
private:
|
|
QNetworkAccessManager* m_nam;
|
|
};
|
|
|
|
int main(int argc, char* argv[])
|
|
{
|
|
QApplication app(argc, argv);
|
|
app.setApplicationName(QStringLiteral("kdeconnectd"));
|
|
app.setApplicationVersion(QStringLiteral(KDECONNECT_VERSION_STRING));
|
|
app.setOrganizationDomain(QStringLiteral("kde.org"));
|
|
app.setQuitOnLastWindowClosed(false);
|
|
|
|
KDBusService dbusService(KDBusService::Unique);
|
|
|
|
Daemon* daemon = new DesktopDaemon;
|
|
QObject::connect(daemon, SIGNAL(destroyed(QObject*)), &app, SLOT(quit()));
|
|
|
|
return app.exec();
|
|
}
|
|
|
|
#include "kdeconnectd.moc"
|