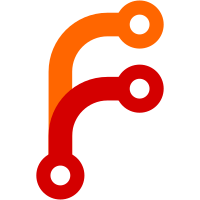
It's more expressive in the sense that it makes clear that the user should not delete the object
127 lines
3.9 KiB
C++
127 lines
3.9 KiB
C++
/**
|
|
* Copyright 2015 Vineet Garg <grgvineet@gmail.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License or (at your option) version 3 or any later version
|
|
* accepted by the membership of KDE e.V. (or its successor approved
|
|
* by the membership of KDE e.V.), which shall act as a proxy
|
|
* defined in Section 14 of version 3 of the license.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include "../core/device.h"
|
|
#include "../core/backends/lan/lanlinkprovider.h"
|
|
#include "../core/kdeconnectconfig.h"
|
|
|
|
#include <QtTest>
|
|
|
|
/**
|
|
* This class tests the working of device class
|
|
*/
|
|
class DeviceTest : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
private Q_SLOTS:
|
|
|
|
void initTestCase();
|
|
void testUnpairedDevice();
|
|
void testPairedDevice();
|
|
void cleanupTestCase();
|
|
|
|
private:
|
|
QString deviceId;
|
|
QString deviceName;
|
|
QString deviceType;
|
|
NetworkPacket* identityPacket;
|
|
|
|
};
|
|
|
|
void DeviceTest::initTestCase()
|
|
{
|
|
deviceId = QStringLiteral("testdevice");
|
|
deviceName = QStringLiteral("Test Device");
|
|
deviceType = QStringLiteral("smartphone");
|
|
QString stringPacket = QStringLiteral("{\"id\":1439365924847,\"type\":\"kdeconnect.identity\",\"body\":{\"deviceId\":\"testdevice\",\"deviceName\":\"Test Device\",\"protocolVersion\":6,\"deviceType\":\"phone\"}}");
|
|
identityPacket = new NetworkPacket(QStringLiteral("kdeconnect.identity"));
|
|
NetworkPacket::unserialize(stringPacket.toLatin1(), identityPacket);
|
|
}
|
|
|
|
void DeviceTest::testPairedDevice()
|
|
{
|
|
KdeConnectConfig::instance().addTrustedDevice(deviceId, deviceName, deviceType);
|
|
KdeConnectConfig::instance().setDeviceProperty(deviceId, QStringLiteral("certificate"), QString::fromLatin1(KdeConnectConfig::instance().certificate().toPem())); // Using same certificate from kcc, instead of generating one
|
|
|
|
Device device(this, deviceId);
|
|
|
|
QCOMPARE(device.id(), deviceId);
|
|
QCOMPARE(device.name(), deviceName);
|
|
QCOMPARE(device.type(), deviceType);
|
|
|
|
QCOMPARE(device.isTrusted(), true);
|
|
|
|
QCOMPARE(device.isReachable(), false);
|
|
|
|
// Add link
|
|
LanLinkProvider linkProvider;
|
|
QSslSocket socket;
|
|
LanDeviceLink* link = new LanDeviceLink(deviceId, &linkProvider, &socket, LanDeviceLink::Locally);
|
|
device.addLink(*identityPacket, link);
|
|
|
|
QCOMPARE(device.isReachable(), true);
|
|
QCOMPARE(device.availableLinks().contains(linkProvider.name()), true);
|
|
|
|
// Remove link
|
|
device.removeLink(link);
|
|
|
|
QCOMPARE(device.isReachable(), false);
|
|
QCOMPARE(device.availableLinks().contains(linkProvider.name()), false);
|
|
|
|
device.unpair();
|
|
QCOMPARE(device.isTrusted(), false);
|
|
|
|
}
|
|
|
|
void DeviceTest::testUnpairedDevice()
|
|
{
|
|
KdeConnectConfig::instance().removeTrustedDevice(deviceId);
|
|
|
|
LanLinkProvider linkProvider;
|
|
QSslSocket socket;
|
|
LanDeviceLink* link = new LanDeviceLink(deviceId, &linkProvider, &socket, LanDeviceLink::Locally);
|
|
|
|
Device device(this, *identityPacket, link);
|
|
|
|
QCOMPARE(device.id(), deviceId);
|
|
QCOMPARE(device.name(), deviceName);
|
|
QCOMPARE(device.type(), deviceType);
|
|
|
|
QCOMPARE(device.isTrusted(), false);
|
|
|
|
QCOMPARE(device.isReachable(), true);
|
|
QCOMPARE(device.availableLinks().contains(linkProvider.name()), true);
|
|
|
|
// Remove link
|
|
device.removeLink(link);
|
|
|
|
QCOMPARE(device.isReachable(), false);
|
|
QCOMPARE(device.availableLinks().contains(linkProvider.name()), false);
|
|
}
|
|
|
|
void DeviceTest::cleanupTestCase()
|
|
{
|
|
delete identityPacket;
|
|
}
|
|
|
|
QTEST_GUILESS_MAIN(DeviceTest)
|
|
|
|
#include "devicetest.moc"
|