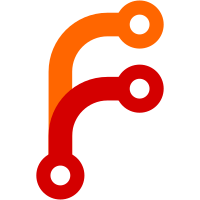
When sharing a file, add metadata about the file's creation time and last modified time. When receiving a shared file, read those fields and set them on the file we create. Note: Qt doesn't support setting the creation date on Unix [1]. [1] https://code.qt.io/cgit/qt/qtbase.git/tree/src/corelib/io/qfilesystemengine_unix.cpp?h=v5.15.2&id=40143c189b7c1bf3c2058b77d00ea5c4e3be8b28#n1590
53 lines
1.7 KiB
C++
53 lines
1.7 KiB
C++
/**
|
|
* SPDX-FileCopyrightText: 2013 Albert Vaca <albertvaka@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-only OR GPL-3.0-only OR LicenseRef-KDE-Accepted-GPL
|
|
*/
|
|
|
|
#ifndef SHAREPLUGIN_H
|
|
#define SHAREPLUGIN_H
|
|
|
|
#include <QPointer>
|
|
|
|
#include <core/kdeconnectplugin.h>
|
|
#include <core/compositefiletransferjob.h>
|
|
|
|
#define PACKET_TYPE_SHARE_REQUEST QStringLiteral("kdeconnect.share.request")
|
|
#define PACKET_TYPE_SHARE_REQUEST_UPDATE QStringLiteral("kdeconnect.share.request.update")
|
|
|
|
class SharePlugin
|
|
: public KdeConnectPlugin
|
|
{
|
|
Q_OBJECT
|
|
Q_CLASSINFO("D-Bus Interface", "org.kde.kdeconnect.device.share")
|
|
|
|
public:
|
|
explicit SharePlugin(QObject* parent, const QVariantList& args);
|
|
|
|
///Helper method, QDBus won't recognize QUrl
|
|
Q_SCRIPTABLE void shareUrl(const QString& url) { shareUrl(QUrl(url), false); }
|
|
Q_SCRIPTABLE void shareUrls(const QStringList& urls);
|
|
Q_SCRIPTABLE void shareText(const QString& text);
|
|
Q_SCRIPTABLE void openFile(const QString& file) { shareUrl(QUrl(file), true); }
|
|
|
|
bool receivePacket(const NetworkPacket& np) override;
|
|
void connected() override {}
|
|
QString dbusPath() const override;
|
|
|
|
private Q_SLOTS:
|
|
void openDestinationFolder();
|
|
|
|
Q_SIGNALS:
|
|
Q_SCRIPTABLE void shareReceived(const QString& url);
|
|
|
|
private:
|
|
void finished(KJob* job, const qint64 dateCreated, const qint64 dateModified, const bool open);
|
|
void shareUrl(const QUrl& url, bool open);
|
|
QUrl destinationDir() const;
|
|
QUrl getFileDestination(const QString filename) const;
|
|
void setDateModified(const QUrl& destination, const qint64 timestamp);
|
|
void setDateCreated(const QUrl& destination, const qint64 timestamp);
|
|
|
|
QPointer<CompositeFileTransferJob> m_compositeJob;
|
|
};
|
|
#endif
|