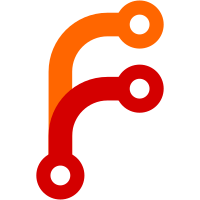
## Summary This adds character counter below the “Send” button in SMS conversation. It uses format XXX/Y where XXX is number of characters that can be added without splitting the SMS into multiple messages (see article Concatenated SMS on Wikipedia). Y is number of messages in in current concatenated SMS. The counter is not visible when insertion of 10 7-bit or 16-bit (depends on SMS encoding) does not create concatenated SMS. SMS encoding is automatically guessed. 8-bit encodings are not supported. If the message contains characters that are not supported by GSM 7-bit encoding, counter automatically switches to UCS-2. ## Test Plan Try entering some text that is longer than 150 characters in [GSM 03.38 encoding][1] or 60 characters in UCS-2. Number of remaining characters should be visible below the “Send” button. The character counter should show `0` at exactly 160 or 70 characters. Inserting one character should switch the counter to [Concatenated SMS][2] mode when number of messages is shown. It should show exactly same number as SMS app in Android. ## Screenshots These images are in APNG.   [1]: https://en.wikipedia.org/w/index.php?oldid=932080074#GSM_7-bit_default_alphabet_and_extension_table_of_3GPP_TS_23.038_/_GSM_03.38 [2]: https://en.wikipedia.org/w/index.php?oldid=943185255#Message_size
154 lines
4 KiB
C++
154 lines
4 KiB
C++
/**
|
|
* Copyright (C) 2020 Jiří Wolker <woljiri@gmail.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License or (at your option) version 3 or any later version
|
|
* accepted by the membership of KDE e.V. (or its successor approved
|
|
* by the membership of KDE e.V.), which shall act as a proxy
|
|
* defined in Section 14 of version 3 of the license.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
/**
|
|
* Map used to determine that ASCII character is in GSM 03.38 7-bit alphabet.
|
|
*
|
|
* Only allowed control characters are CR and LF but GSM alphabet has more of them.
|
|
*/
|
|
bool gsm_ascii_map[] = {
|
|
false, // 0x0, some control char
|
|
false, // 0x1, some control char
|
|
false, // 0x2, some control char
|
|
false, // 0x3, some control char
|
|
false, // 0x4, some control char
|
|
false, // 0x5, some control char
|
|
false, // 0x6, some control char
|
|
false, // 0x7, some control char
|
|
false, // 0x8, some control char
|
|
false, // 0x9, some control char
|
|
true, // 0xA, LF
|
|
false, // 0xB, some control char
|
|
false, // 0xC, some control char
|
|
true, // 0xD, CR
|
|
false, // 0xE, some control char
|
|
false, // 0xF, some control char
|
|
false, // 0x10, some control char
|
|
false, // 0x11, some control char
|
|
false, // 0x12, some control char
|
|
false, // 0x13, some control char
|
|
false, // 0x14, some control char
|
|
false, // 0x15, some control char
|
|
false, // 0x16, some control char
|
|
false, // 0x17, some control char
|
|
false, // 0x18, some control char
|
|
false, // 0x19, some control char
|
|
false, // 0x1A, some control char
|
|
false, // 0x1B, some control char
|
|
false, // 0x1C, some control char
|
|
false, // 0x1D, some control char
|
|
false, // 0x1E, some control char
|
|
false, // 0x1F, some control char
|
|
true, // 20, space
|
|
true, // 21, !
|
|
true, // 22, "
|
|
true, // 23, #
|
|
true, // 24, $
|
|
true, // 25, %
|
|
true, // 26, &
|
|
true, // 27, '
|
|
true, // 28, (
|
|
true, // 29, )
|
|
true, // 2A, *
|
|
true, // 2B, +
|
|
true, // 2C, ,
|
|
true, // 2D, -
|
|
true, // 2E, .
|
|
true, // 2F, /
|
|
true, // 30, 0
|
|
true, // 31, 1
|
|
true, // 32, 2
|
|
true, // 33, 3
|
|
true, // 34, 4
|
|
true, // 35, 5
|
|
true, // 36, 6
|
|
true, // 37, 7
|
|
true, // 38, 8
|
|
true, // 39, 9
|
|
true, // 3A, :
|
|
true, // 3B, ;
|
|
true, // 3C, <
|
|
true, // 3D, =
|
|
true, // 3E, >
|
|
true, // 3F, ?
|
|
true, // 40, @
|
|
true, // 41, A
|
|
true, // 42, B
|
|
true, // 43, C
|
|
true, // 44, D
|
|
true, // 45, E
|
|
true, // 46, F
|
|
true, // 47, G
|
|
true, // 48, H
|
|
true, // 49, I
|
|
true, // 4A, J
|
|
true, // 4B, K
|
|
true, // 4C, L
|
|
true, // 4D, M
|
|
true, // 4E, N
|
|
true, // 4F, O
|
|
true, // 50, P
|
|
true, // 51, Q
|
|
true, // 52, R
|
|
true, // 53, S
|
|
true, // 54, T
|
|
true, // 55, U
|
|
true, // 56, V
|
|
true, // 57, W
|
|
true, // 58, X
|
|
true, // 59, Y
|
|
true, // 5A, Z
|
|
false, // 5B, [
|
|
false, // 5C, backslash
|
|
false, // 5D, ]
|
|
false, // 5E, ^
|
|
true, // 5F, _
|
|
false, // 60, `
|
|
true, // 61, a
|
|
true, // 62, b
|
|
true, // 63, c
|
|
true, // 64, d
|
|
true, // 65, e
|
|
true, // 66, f
|
|
true, // 67, g
|
|
true, // 68, h
|
|
true, // 69, i
|
|
true, // 6A, j
|
|
true, // 6B, k
|
|
true, // 6C, l
|
|
true, // 6D, m
|
|
true, // 6E, n
|
|
true, // 6F, o
|
|
true, // 70, p
|
|
true, // 71, q
|
|
true, // 72, r
|
|
true, // 73, s
|
|
true, // 74, t
|
|
true, // 75, u
|
|
true, // 76, v
|
|
true, // 77, w
|
|
true, // 78, x
|
|
true, // 79, y
|
|
true, // 7A, z
|
|
false, // 7B, {
|
|
false, // 7C, |
|
|
false, // 7D, }
|
|
false, // 7E, ~
|
|
};
|