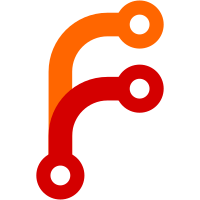
Summary: When a new message is delivered, the conversation list should update by changing the preview text and re-sorting the conversations Bonus bug discovered and fixed: previously, when the conversations list was being populated, it made a request for the first message in every conversation. This would be fine if the conversationdbusinterface pulled from local cache. However, this actually triggers a request to the phone for *every* conversation. This should be handled differently in conversationdbusinterface's requestConversation as well, but that's a project for a later day (TODO comments added) Test Plan: - Launch SMS app - Verify conversations list appears - Verify lack of massive stream of debug output indicating lots of messages for the wrong conversation are being received - Verify that opening a particular conversation shows the messages after a short delay while the backend fetches the content from the phone - Verify that receiving a new message into an existing conversation updates the conversation list Reviewers: #kde_connect, nicolasfella Reviewed By: #kde_connect, nicolasfella Subscribers: nicolasfella, apol, kdeconnect Tags: #kde_connect Differential Revision: https://phabricator.kde.org/D15608
118 lines
3.4 KiB
C++
118 lines
3.4 KiB
C++
/*
|
|
* This file is part of KDE Telepathy Chat
|
|
*
|
|
* Copyright (C) 2018 Aleix Pol Gonzalez <aleixpol@kde.org>
|
|
* Copyright (C) 2018 Simon Redman <simon@ergotech.com>
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
#ifndef CONVERSATIONLISTMODEL_H
|
|
#define CONVERSATIONLISTMODEL_H
|
|
|
|
#include <QStandardItemModel>
|
|
#include <QLoggingCategory>
|
|
#include <QQmlParserStatus>
|
|
#include <KPeople/kpeople/personsmodel.h>
|
|
#include <KPeople/kpeople/persondata.h>
|
|
|
|
#include "interfaces/conversationmessage.h"
|
|
#include "interfaces/dbusinterfaces.h"
|
|
|
|
Q_DECLARE_LOGGING_CATEGORY(KDECONNECT_SMS_CONVERSATIONS_LIST_MODEL)
|
|
|
|
|
|
class OurSortFilterProxyModel : public QSortFilterProxyModel, public QQmlParserStatus
|
|
{
|
|
Q_OBJECT
|
|
Q_INTERFACES(QQmlParserStatus)
|
|
Q_PROPERTY(Qt::SortOrder sortOrder READ sortOrder WRITE setSortOrder)
|
|
public:
|
|
|
|
Qt::SortOrder sortOrder() const { return m_sortOrder; }
|
|
void setSortOrder(Qt::SortOrder sortOrder) {
|
|
if (m_sortOrder != sortOrder) {
|
|
m_sortOrder = sortOrder;
|
|
sortNow();
|
|
}
|
|
}
|
|
void classBegin() override {}
|
|
void componentComplete() override {
|
|
m_completed = true;
|
|
sortNow();
|
|
}
|
|
|
|
private:
|
|
void sortNow() {
|
|
if (m_completed && dynamicSortFilter())
|
|
sort(0, m_sortOrder);
|
|
}
|
|
|
|
bool m_completed = false;
|
|
Qt::SortOrder m_sortOrder = Qt::AscendingOrder;
|
|
};
|
|
|
|
class ConversationListModel
|
|
: public QStandardItemModel
|
|
{
|
|
Q_OBJECT
|
|
Q_PROPERTY(QString deviceId READ deviceId WRITE setDeviceId)
|
|
|
|
public:
|
|
ConversationListModel(QObject* parent = nullptr);
|
|
~ConversationListModel();
|
|
|
|
enum Roles {
|
|
FromMeRole = Qt::UserRole,
|
|
PersonUriRole,
|
|
AddressRole,
|
|
ConversationIdRole,
|
|
DateRole,
|
|
};
|
|
Q_ENUM(Roles)
|
|
|
|
QString deviceId() const { return m_deviceId; }
|
|
void setDeviceId(const QString &/*deviceId*/);
|
|
|
|
public Q_SLOTS:
|
|
void handleCreatedConversation(const QVariantMap& msg);
|
|
void handleConversationUpdated(const QVariantMap& msg);
|
|
void createRowFromMessage(const QVariantMap& message);
|
|
void printDBusError(const QDBusError& error);
|
|
|
|
private:
|
|
/**
|
|
* Get all conversations currently known by the conversationsInterface, if any
|
|
*/
|
|
void prepareConversationsList();
|
|
|
|
/**
|
|
* Get the data for a particular person given their contact address
|
|
*/
|
|
KPeople::PersonData* lookupPersonByAddress(const QString& address);
|
|
|
|
/**
|
|
* Simplify a phone number to a known form
|
|
*/
|
|
QString canonicalizePhoneNumber(const QString& phoneNumber);
|
|
|
|
QStandardItem* conversationForThreadId(qint32 threadId);
|
|
|
|
DeviceConversationsDbusInterface* m_conversationsInterface;
|
|
QString m_deviceId;
|
|
KPeople::PersonsModel m_people;
|
|
};
|
|
|
|
#endif // CONVERSATIONLISTMODEL_H
|