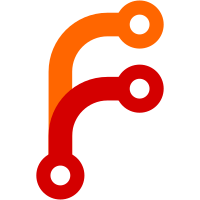
Now that devices can potentially be connected via both network and bluetooth simultaneously we should prioritise connections over the highest performing link (probably wifi/network). To this end the m_deviceLinks are now sorted based on priority with the fastest links first; this means that when Device::sendPacket is scheduling to send a packet, it should always use the fastest link first.
44 lines
786 B
C++
44 lines
786 B
C++
/**
|
|
* SPDX-FileCopyrightText: 2013 Albert Vaca <albertvaka@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-only OR GPL-3.0-only OR LicenseRef-KDE-Accepted-GPL
|
|
*/
|
|
|
|
#ifndef DEVICELINK_H
|
|
#define DEVICELINK_H
|
|
|
|
#include <QObject>
|
|
|
|
#include "deviceinfo.h"
|
|
#include "networkpacket.h"
|
|
|
|
class LinkProvider;
|
|
|
|
class DeviceLink : public QObject
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
DeviceLink(const QString &deviceId, LinkProvider *parent);
|
|
|
|
QString deviceId() const
|
|
{
|
|
return deviceInfo().id;
|
|
}
|
|
|
|
int priority() const
|
|
{
|
|
return priorityFromProvider;
|
|
}
|
|
|
|
virtual bool sendPacket(NetworkPacket &np) = 0;
|
|
|
|
virtual DeviceInfo deviceInfo() const = 0;
|
|
|
|
private:
|
|
int priorityFromProvider;
|
|
|
|
Q_SIGNALS:
|
|
void receivedPacket(const NetworkPacket &np);
|
|
};
|
|
|
|
#endif
|