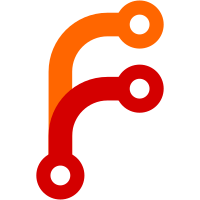
The rationale is explained in https://planet.kde.org/friedrich-kossebau-2023-06-28-include-also-moc-files-of-headers/ In case of KDEConnect, it impressively speeds up compilation. Before it took 390 seconds on a clean build and with this change it took 330 seconds. This is due to the mocs_compilation having to include the header files and thus all their headers. Due to the lots of small plugins we have, this means that the same headers must be compiled plenty of times. When we include the moc files directly in the C++ file, they are already available.
117 lines
3.9 KiB
C++
117 lines
3.9 KiB
C++
/**
|
|
* SPDX-FileCopyrightText: 2013 Albert Vaca <albertvaka@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-only OR GPL-3.0-only OR LicenseRef-KDE-Accepted-GPL
|
|
*/
|
|
|
|
#include "landevicelink.h"
|
|
|
|
#include <KLocalizedString>
|
|
|
|
#include "backends/linkprovider.h"
|
|
#include "core_debug.h"
|
|
#include "kdeconnectconfig.h"
|
|
#include "lanlinkprovider.h"
|
|
#include "plugins/share/shareplugin.h"
|
|
|
|
LanDeviceLink::LanDeviceLink(const DeviceInfo &deviceInfo, LanLinkProvider *parent, QSslSocket *socket)
|
|
: DeviceLink(deviceInfo.id, parent)
|
|
, m_socket(nullptr)
|
|
, m_deviceInfo(deviceInfo)
|
|
{
|
|
reset(socket);
|
|
}
|
|
|
|
void LanDeviceLink::reset(QSslSocket *socket)
|
|
{
|
|
if (m_socket) {
|
|
disconnect(m_socket, &QAbstractSocket::disconnected, this, &QObject::deleteLater);
|
|
delete m_socket;
|
|
}
|
|
|
|
m_socket = socket;
|
|
socket->setParent(this);
|
|
|
|
connect(socket, &QAbstractSocket::disconnected, this, &QObject::deleteLater);
|
|
connect(socket, &QAbstractSocket::readyRead, this, &LanDeviceLink::dataReceived);
|
|
}
|
|
|
|
QHostAddress LanDeviceLink::hostAddress() const
|
|
{
|
|
if (!m_socket) {
|
|
return QHostAddress::Null;
|
|
}
|
|
QHostAddress addr = m_socket->peerAddress();
|
|
if (addr.protocol() == QAbstractSocket::IPv6Protocol) {
|
|
bool success;
|
|
QHostAddress convertedAddr = QHostAddress(addr.toIPv4Address(&success));
|
|
if (success) {
|
|
qCDebug(KDECONNECT_CORE) << "Converting IPv6" << addr << "to IPv4" << convertedAddr;
|
|
addr = convertedAddr;
|
|
}
|
|
}
|
|
return addr;
|
|
}
|
|
|
|
bool LanDeviceLink::sendPacket(NetworkPacket &np)
|
|
{
|
|
if (np.payload()) {
|
|
if (np.type() == PACKET_TYPE_SHARE_REQUEST && np.payloadSize() >= 0) {
|
|
if (!m_compositeUploadJob || !m_compositeUploadJob->isRunning()) {
|
|
m_compositeUploadJob = new CompositeUploadJob(deviceId(), true);
|
|
}
|
|
|
|
m_compositeUploadJob->addSubjob(new UploadJob(np));
|
|
|
|
if (!m_compositeUploadJob->isRunning()) {
|
|
m_compositeUploadJob->start();
|
|
}
|
|
} else { // Infinite stream
|
|
CompositeUploadJob *fireAndForgetJob = new CompositeUploadJob(deviceId(), false);
|
|
fireAndForgetJob->addSubjob(new UploadJob(np));
|
|
fireAndForgetJob->start();
|
|
}
|
|
|
|
return true;
|
|
} else {
|
|
int written = m_socket->write(np.serialize());
|
|
|
|
// Actually we can't detect if a packet is received or not. We keep TCP
|
|
//"ESTABLISHED" connections that look legit (return true when we use them),
|
|
// but that are actually broken (until keepalive detects that they are down).
|
|
return (written != -1);
|
|
}
|
|
}
|
|
|
|
void LanDeviceLink::dataReceived()
|
|
{
|
|
while (m_socket->canReadLine()) {
|
|
const QByteArray serializedPacket = m_socket->readLine();
|
|
NetworkPacket packet;
|
|
NetworkPacket::unserialize(serializedPacket, &packet);
|
|
|
|
// qCDebug(KDECONNECT_CORE) << "LanDeviceLink dataReceived" << serializedPacket;
|
|
|
|
if (packet.hasPayloadTransferInfo()) {
|
|
// qCDebug(KDECONNECT_CORE) << "HasPayloadTransferInfo";
|
|
const QVariantMap transferInfo = packet.payloadTransferInfo();
|
|
|
|
QSharedPointer<QSslSocket> socket(new QSslSocket);
|
|
|
|
LanLinkProvider::configureSslSocket(socket.data(), deviceId(), true);
|
|
|
|
// emit readChannelFinished when the socket gets disconnected. This seems to be a bug in upstream QSslSocket.
|
|
// Needs investigation and upstreaming of the fix. QTBUG-62257
|
|
connect(socket.data(), &QAbstractSocket::disconnected, socket.data(), &QAbstractSocket::readChannelFinished);
|
|
|
|
const QString address = m_socket->peerAddress().toString();
|
|
const quint16 port = transferInfo[QStringLiteral("port")].toInt();
|
|
socket->connectToHostEncrypted(address, port, QIODevice::ReadWrite);
|
|
packet.setPayload(socket, packet.payloadSize());
|
|
}
|
|
|
|
Q_EMIT receivedPacket(packet);
|
|
}
|
|
}
|
|
|
|
#include "moc_landevicelink.cpp"
|