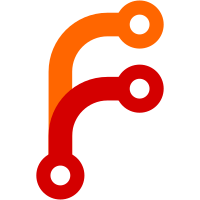
Summary: Change all member variables to the form m_fooBar because it is the preferred form in Qt (it was half and half between this and mFooBar, and a minority didn't have anything). Place all references and pointers on the side of the type since it is the majority. Basically: - mFoo -> m_foo - foo -> m_foo (if it is a member variable) - Type &ref -> Type& ref - Type *ptr -> Type* ptr Reviewers: #kde_connect, nicolasfella, albertvaka Reviewed By: #kde_connect, nicolasfella, albertvaka Subscribers: albertvaka, #kde_connect Tags: #kde_connect Differential Revision: https://phabricator.kde.org/D7312
61 lines
1.3 KiB
C++
61 lines
1.3 KiB
C++
|
|
#ifndef RESPONSE_WAITER_H
|
|
#define RESPONSE_WAITER_H
|
|
|
|
#include <QObject>
|
|
#include <QVariant>
|
|
#include <QTimer>
|
|
|
|
class QDBusPendingCall;
|
|
class QDBusPendingCallWatcher;
|
|
|
|
class DBusResponseWaiter : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
|
|
static DBusResponseWaiter* instance();
|
|
|
|
///extract QDbusPendingCall from \p variant and blocks untill completed
|
|
Q_INVOKABLE QVariant waitForReply(QVariant variant) const;
|
|
|
|
const QDBusPendingCall* extractPendingCall(QVariant& variant) const;
|
|
|
|
private:
|
|
DBusResponseWaiter();
|
|
|
|
static DBusResponseWaiter* m_instance;
|
|
QList<int> m_registered;
|
|
};
|
|
|
|
|
|
class DBusAsyncResponse : public QObject
|
|
{
|
|
Q_OBJECT
|
|
Q_PROPERTY(bool autoDelete READ autodelete WRITE setAutodelete)
|
|
|
|
public:
|
|
explicit DBusAsyncResponse(QObject* parent = nullptr);
|
|
~DBusAsyncResponse() override = default;
|
|
|
|
Q_INVOKABLE void setPendingCall(QVariant e);
|
|
|
|
void setAutodelete(bool b) {m_autodelete = b;};
|
|
bool autodelete() const {return m_autodelete;}
|
|
|
|
Q_SIGNALS:
|
|
void success(const QVariant& result);
|
|
void error(const QString& message);
|
|
|
|
private Q_SLOTS:
|
|
void onCallFinished(QDBusPendingCallWatcher* watcher);
|
|
void onTimeout();
|
|
|
|
private:
|
|
QTimer m_timeout;
|
|
bool m_autodelete;
|
|
};
|
|
|
|
|
|
#endif
|