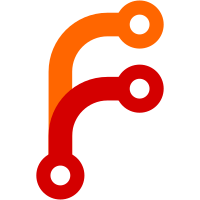
## Summary Add support for Phone side (like in the Android and SailfishOS apps) to telephony plugin. this requires ModemManager to work. Call state notifications are currently supported, more capabilities are possible. Tested on PinePhone but should probably work on every linux Phone with ModemManager. to test, pair kdeconnect on your linux phone to desktop, and call your phone. you should get notification on the desktop, telling you that phone call is incoming.
57 lines
1.7 KiB
C++
57 lines
1.7 KiB
C++
/**
|
|
* SPDX-FileCopyrightText: 2013 Albert Vaca <albertvaka@gmail.com>
|
|
* SPDX-FileCopyrightText: 2018 Simon Redman <simon@ergotech.com>
|
|
* SPDX-FileCopyrightText: 2019 Richard Liebscher <richard.liebscher@gmail.com>
|
|
* SPDX-FileCopyrightText: 2022 Yoram Bar Haim <bhyoram@protonmail.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-only OR GPL-3.0-only OR LicenseRef-KDE-Accepted-GPL
|
|
*/
|
|
|
|
#ifndef TELEPHONYPLUGIN_H
|
|
#define TELEPHONYPLUGIN_H
|
|
|
|
#include <KPluginFactory>
|
|
#include <ModemManagerQt/Call>
|
|
#include <ModemManagerQt/Manager>
|
|
#include <ModemManagerQt/Modem>
|
|
#include <ModemManagerQt/ModemVoice>
|
|
#include <QList>
|
|
#include <QMap>
|
|
#include <QObject>
|
|
#include <QSet>
|
|
#include <QVariant>
|
|
|
|
#include <core/kdeconnectplugin.h>
|
|
|
|
class QDBusPendingCallWatcher;
|
|
class QDBusInterface;
|
|
class QDBusError;
|
|
class QDBusObjectPath;
|
|
class QDBusVariant;
|
|
|
|
class MMTelephonyPlugin : public KdeConnectPlugin
|
|
{
|
|
Q_OBJECT
|
|
Q_CLASSINFO("D-Bus Interface", "org.kde.kdeconnect.device.telephony")
|
|
|
|
public:
|
|
explicit MMTelephonyPlugin(QObject *parent, const QVariantList &args);
|
|
~MMTelephonyPlugin() override = default;
|
|
|
|
bool receivePacket(const NetworkPacket &np) override;
|
|
void connected() override
|
|
{
|
|
}
|
|
|
|
private:
|
|
void onCallAdded(ModemManager::Call::Ptr call);
|
|
void onCallRemoved(ModemManager::Call::Ptr call);
|
|
void onModemAdded(const QString &path);
|
|
void onModemRemoved(const QString &path);
|
|
void onCallStateChanged(ModemManager::Call *call, MMCallState newState, MMCallState oldState, MMCallStateReason reason);
|
|
void sendMMTelephonyPacket(ModemManager::Call *call, const QString &state);
|
|
void sendCancelMMTelephonyPacket(ModemManager::Call *call, const QString &lastState);
|
|
static QString stateName(MMCallState state);
|
|
};
|
|
|
|
#endif
|