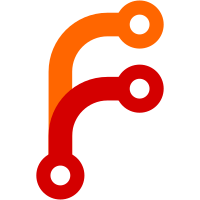
BUG: 485829 ## Summary Currently, the plugin just fails silently if the local device is missing the `krfb` package or if the remote device misses an `vnc://` protocol/scheme handler. You click the button and nothing happens. One issue is, that the plugin is considered `virtualmonitor.available` in the `DeviceDelegate.qml`, even if the check for `krfb-virtualmonitor` fails and no dbus-path is provided. I investigated the behavior a bit, but ignored it in the end as this MR benefits from being shown for device constellations that _could_ provide this feature. A warning is shown with brief instructions, how to get the plugin working correctly. - Check if krfb-virtualmonitor is available locally - Check default scheme handler for vnc:// on device (Linux) - Show warnings / reasons, if no connection could be established ## Test Plan Regarding if the devices have mentioned packages installed, we should see different behaviors. If the remote device has no VNC client, it can not connect to out server. _A warning should be shown._ If the local device hasn't the `krfb-virtualmonitor` available, the remote device couldn't connect. _A warning should be shown._ If both problems are present, _both warnings should be shown._ If none of these are present, no warning should be shown and we should try to establish a connection. The connection attempts failed? _A warning should be shown._
64 lines
1.8 KiB
C++
64 lines
1.8 KiB
C++
/**
|
|
* SPDX-FileCopyrightText: 2021 Aleix Pol i Gonzalez <aleixpol@kde.org>
|
|
* SPDX-FileCopyrightText: 2024 Fabian Arndt <fabian.arndt@root-core.net>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-only OR GPL-3.0-only OR LicenseRef-KDE-Accepted-GPL
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include "plugin_virtualmonitor_debug.h"
|
|
#include <QJsonObject>
|
|
#include <core/kdeconnectplugin.h>
|
|
|
|
#define PACKET_TYPE_VIRTUALMONITOR QStringLiteral("kdeconnect.virtualmonitor")
|
|
#define PACKET_TYPE_VIRTUALMONITOR_REQUEST QStringLiteral("kdeconnect.virtualmonitor.request")
|
|
|
|
class QProcess;
|
|
|
|
class VirtualMonitorPlugin : public KdeConnectPlugin
|
|
{
|
|
Q_OBJECT
|
|
Q_CLASSINFO("D-Bus Interface", "org.kde.kdeconnect.device.virtualmonitor")
|
|
Q_PROPERTY(bool hasRemoteVncClient READ hasRemoteVncClient CONSTANT)
|
|
Q_PROPERTY(bool isVirtualMonitorAvailable READ isVirtualMonitorAvailable CONSTANT)
|
|
|
|
public:
|
|
using KdeConnectPlugin::KdeConnectPlugin;
|
|
~VirtualMonitorPlugin() override;
|
|
|
|
Q_SCRIPTABLE bool requestVirtualMonitor();
|
|
|
|
void connected() override;
|
|
QString dbusPath() const override;
|
|
void receivePacket(const NetworkPacket &np) override;
|
|
|
|
// The remote device has a VNC client installed
|
|
bool hasRemoteVncClient() const
|
|
{
|
|
return m_capabilitiesRemote.vncClient;
|
|
}
|
|
|
|
// krfb is installed on local device, virtual monitors is supported
|
|
bool isVirtualMonitorAvailable() const
|
|
{
|
|
return m_capabilitiesLocal.virtualMonitor;
|
|
}
|
|
|
|
private:
|
|
void stop();
|
|
bool checkVncClient() const;
|
|
bool checkDefaultSchemeHandler(const QString &scheme) const;
|
|
|
|
struct Capabilities {
|
|
bool virtualMonitor = false;
|
|
bool vncClient = false;
|
|
};
|
|
|
|
Capabilities m_capabilitiesLocal;
|
|
Capabilities m_capabilitiesRemote;
|
|
|
|
QProcess *m_process = nullptr;
|
|
QJsonObject m_remoteResolution;
|
|
uint m_retries = 0;
|
|
};
|