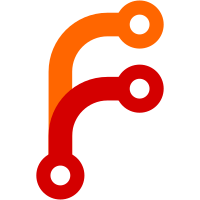
Better patch to replace !218. - Auto and quick detection of previous D-Bus instance; - Remove private D-Bus compile definition, only use it on macOS without an existing D-Bus instance; - Safe reboot after crashes because the indicator is not relating on the kdeconnectd to run a D-Bus session; - Safe exit after clicking on `Quit` in the systray. More details in commit logs: Only enable private D-Bus on macOS because the other platforms do not need them. The app should be able to easily detect the session bus from the env DBUS_LAUNCHD_SESSION_BUS_SOCKET from launchd through launchctl. Because https://gitlab.freedesktop.org/dbus/dbus/-/blob/master/dbus/dbus-sysdeps-unix.c#L4392 shows that it is the only probing method on macOS with launchd. The D-Bus session bus can be easily found from launchd/launchctl with DBUS_LAUNCHD_SESSION_BUS_SOCKET env. It can be an external one (installed from HomeBrew) or an internal one (launched by a previous instance followed by a crash). The indicator helper on macOS can now automatically detect whether we can use a potentially (with launchd/launchctl env set, or KDE Connect macOS private_bus_address set) existed and usable session bus. If previous bus is usable, just try to launch the kdeconnectd with us. Otherwise, launch a private D-Bus daemon, export the launchd/launchctl env, and run a kdeconnectd instance. Everything works better and quicker now :)
81 lines
2 KiB
C++
81 lines
2 KiB
C++
/**
|
|
* SPDX-FileCopyrightText: 2015 Albert Vaca <albertvaka@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-only OR GPL-3.0-only OR LicenseRef-KDE-Accepted-GPL
|
|
*/
|
|
|
|
#ifndef KDECONNECTCONFIG_H
|
|
#define KDECONNECTCONFIG_H
|
|
|
|
#include <QDir>
|
|
|
|
#include "kdeconnectcore_export.h"
|
|
|
|
class QSslCertificate;
|
|
|
|
class KDECONNECTCORE_EXPORT KdeConnectConfig
|
|
{
|
|
public:
|
|
struct DeviceInfo {
|
|
QString deviceName;
|
|
QString deviceType;
|
|
};
|
|
|
|
static KdeConnectConfig& instance();
|
|
|
|
/*
|
|
* Our own info
|
|
*/
|
|
|
|
QString deviceId();
|
|
QString name();
|
|
QString deviceType();
|
|
|
|
QString privateKeyPath();
|
|
QString certificatePath();
|
|
QSslCertificate certificate();
|
|
|
|
void setName(const QString& name);
|
|
|
|
/*
|
|
* Trusted devices
|
|
*/
|
|
|
|
QStringList trustedDevices(); //list of ids
|
|
void removeTrustedDevice(const QString& id);
|
|
void addTrustedDevice(const QString& id, const QString& name, const QString& type);
|
|
KdeConnectConfig::DeviceInfo getTrustedDevice(const QString& id);
|
|
|
|
void setDeviceProperty(const QString& deviceId, const QString& name, const QString& value);
|
|
QString getDeviceProperty(const QString& deviceId, const QString& name, const QString& defaultValue = QString());
|
|
|
|
// Custom devices
|
|
void setCustomDevices(const QStringList& addresses);
|
|
QStringList customDevices() const;
|
|
|
|
/*
|
|
* Paths for config files, there is no guarantee the directories already exist
|
|
*/
|
|
QDir baseConfigDir();
|
|
QDir deviceConfigDir(const QString& deviceId);
|
|
QDir pluginConfigDir(const QString& deviceId, const QString& pluginName); //Used by KdeConnectPluginConfig
|
|
|
|
#ifdef Q_OS_MAC
|
|
/*
|
|
* Get private DBus Address when use private DBus
|
|
*/
|
|
QString privateDBusAddressPath();
|
|
QString privateDBusAddress();
|
|
#endif
|
|
private:
|
|
KdeConnectConfig();
|
|
|
|
void loadPrivateKey();
|
|
void generatePrivateKey(const QString& path);
|
|
void loadCertificate();
|
|
void generateCertificate(const QString& path);
|
|
|
|
struct KdeConnectConfigPrivate* d;
|
|
};
|
|
|
|
#endif
|